A Simple Guide to using OAuth with C#
If you are a newbie to OAuth you might understand how confusing it can be at first! I started off looking at building a small application that consumed an OAuth service as a side project. I just dived right in without understanding how OAuth worked and got myself very confused. I have found that there are loads of examples out there explaining in Ruby, Java and Python - but not that many in C#.
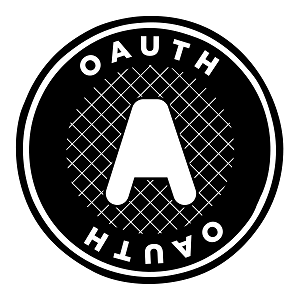
Eventually I found something on StackOverflow that explained everything to me. The answer was well detailed and even included a code sample. This got the ball rolling and I managed to get a working example running. In this article I'll explain a little bit more about OAuth and how simple it really is once you get started.
Firstly, let me start by explaining what OAuth is and why you should use it. OAuth is a simple way to publish and interact with protected data. It is a safer way to give people access to this data when they are calling an API, as each request to the API is signed with encrypted details that only last for a defined duration (e.g. 2 Hours). There are quite a few services out there that use the OAuth standard and some of the big ones are Twitter, Twitpic, Digg and Flickr. There is a good article on the OAuth site that explains more.
I'm not going to go too deep into the whole OAuth process, but I always find that a code sample helps explain things better. Let's take a look at a summarized version of how the process works.
- Register your app with the service that you are developing it for. e.g. Twitter, Twitpic, SoundCloud etc. You will receive a consumer key and secret.
- You, the developer of the app then initiates the OAuth process by passing the consumer key and the consumer secret
- The service will return a Request Token to you.
- The user then needs to grant approval for the app to run requests.
- Once the user has granted permission you need to exchange the request token for an access token.
- Now that you have received an access token, you use this to sign all http requests with your credentials and access token.
I decided to experiment with the Soundcloud API as I regularly check out new music on the site. There is a great wrapper that has already been built for C# OAuth - I used it to connect to the API. Please download it here. Update: Unfortunately this original wrapper I used in this post is no longer available and I suggest using one like this.
To begin the process you need to pass the Consumer Key and Consumer Secret to the service to acquire a Request Token. You will get given this when you register your application with the site.
// Acquire Request Token
OAuth["consumer_key"] = ConsumerKey;
OAuth["consumer_secret"] = ConsumerSecret;
OAuthResponse requestToken = OAuth.AcquireRequestToken("http://api.soundcloud.com/oauth/request_token", "POST");
Now that we have the request token, we need to prompt the user to grant us permission. If you are using a desktop application like I am in this example, use System.Diagnostics.Process to open a new browser window with the URL.
// Start the browser to get our access token
var url = "http://soundcloud.com/oauth/authorize?oauth_token=" + OAuth["token"];
System.Diagnostics.Process.Start(url);
This should open up a window like the following:
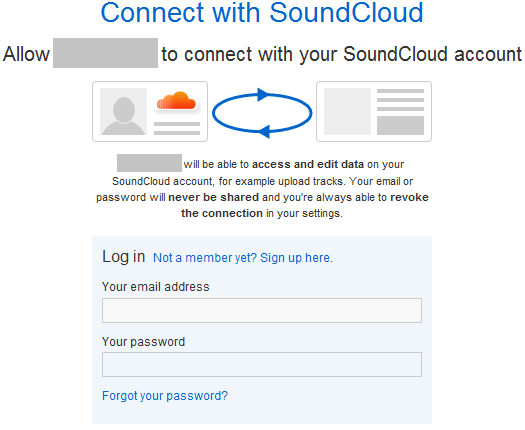
Once the user has logged in they will be given a key that they need to paste back into your application.
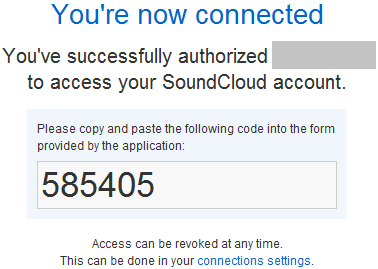
Now, as many users out there might not really be savvy enough to actually do this - the answer on Stackoverflow has a good example of how to bypass this. The article explains an idea that involves some HTML screen scraping to grab the pin. You could use this to copy the pin and use it in your application. This way the user only really needs to authenticate and your app will do the rest. In this example I am just copying the pin and pasting into the Quick Watch window in Visual Studio.
Then we need to exchange the Request Token for an Access Token.
// Acquire access token - prompt user for pin
string pin = string.Empty;
OAuthResponse accessToken = OAuth.AcquireAccessToken("http://api.soundcloud.com/oauth/access_token", "POST", pin);
The Access token is used to sign the HTTP request in the Header.
// Register the app
var search = "http://api.soundcloud.com/me";
var authzHeader = OAuth.GenerateAuthzHeader(search, "GET");
Now that we have the header, we can start requesting information. Pass the information to a GET HTTP request with the Content Type set to "x-www-form-urlencoded" and the Header set as our Authorization Header that we just created. I've bundled this into a separate method as we are going to be using this repeatedly if we need to make other calls to the service.
public string Get(Uri requestUri, string authHeaders) {
if (requestUri == null) { throw new ArgumentNullException("requestUri"); }
HttpWebRequest request = (HttpWebRequest) WebRequest.Create(requestUri);
request.Method = "GET";
request.ServicePoint.Expect100Continue = false;
request.ContentType = "x-www-form-urlencoded";
// Add OAuth headers
request.Headers["Authorization"] = authHeaders;
HttpWebResponse response = (HttpWebResponse) request.GetResponse();
return ReadHttpResponseString(response);
}
That's it! We are now calling a secure service to get the data.
Here are some further links explaining more about OAuth:
And more on the SoundCloud API:
https://github.com/soundcloud/api/wiki/